How many times have you wished you could get the answers or know the contents of a PDF file without opening it? Now, with the influx of tools with AI features, it is possible and as easy as asking a question. You must have seen lots of talk-to-PDF or chat-with-PDF apps popping up recently.
But there’s a catch!
The Chat-to-PDF or talk-to-PDF apps are costly. They tend to provide a few features for a lot of money. Hence, we will show you how to build a talk-toPDF of your own for pretty much free.
For that, we need to spend some time building it. This tutorial will show you how to create your own app using Replit, OpenAI, and an online tool known as LlamaIndex.
By the end of this tutorial, you’ll learn how to:
- Build an app on Replit that uses LlamaIndex and give it a test run.
- Use Replit's AI copilot to modify the code, allowing you to ask your own questions.
- Design a web interface for the app to improve its usability.
Before starting this tutorial, you’ll need:
- Replit account (signup is easy and free!)
- OpenAI platform with $10 in credit. This is separate from your ChatGPT subscription.
Let’s get started. Shall we?
Step 1 - Build a Replit app that uses Llamaindex
The first tool you need is Replit. Go to Replit.com and sign up for an account. Click the ‘Create Repl’ button at the top right corner of the dashboard.
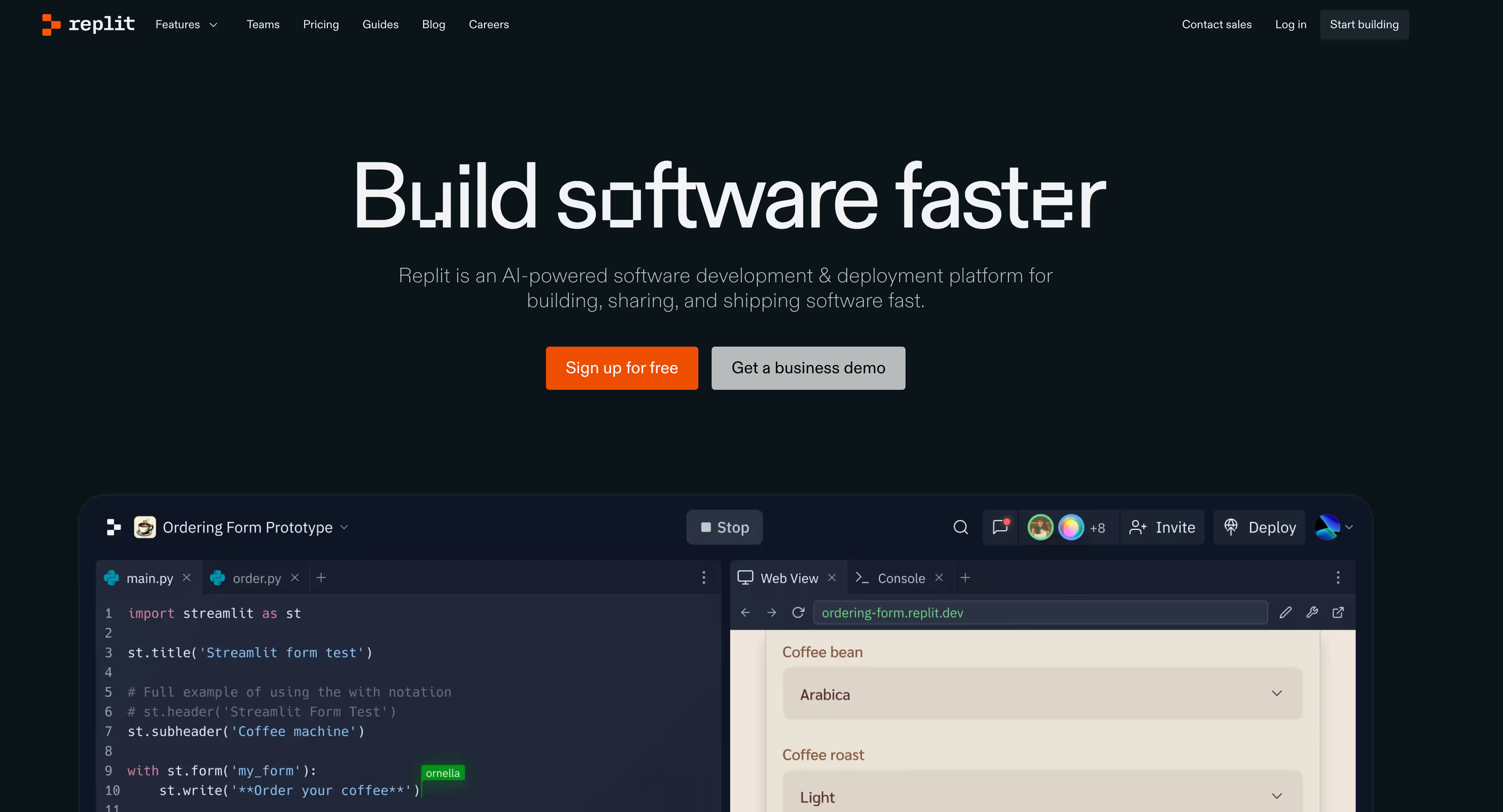
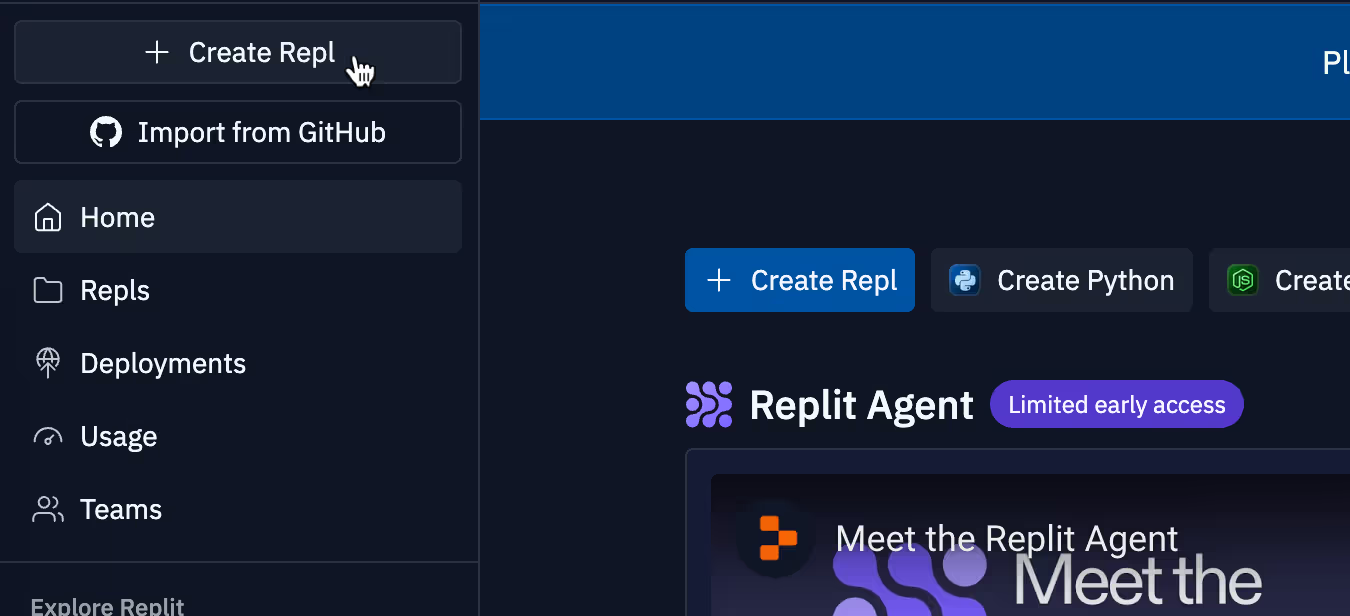
In the ensuing panel, select ‘Python’ from the template drop-down and write a catchy title for your first Repl. You cannot make your app private on a free account; you have to upgrade to do so. Click that cute blue button at the bottom that says, ‘Create Repl.’
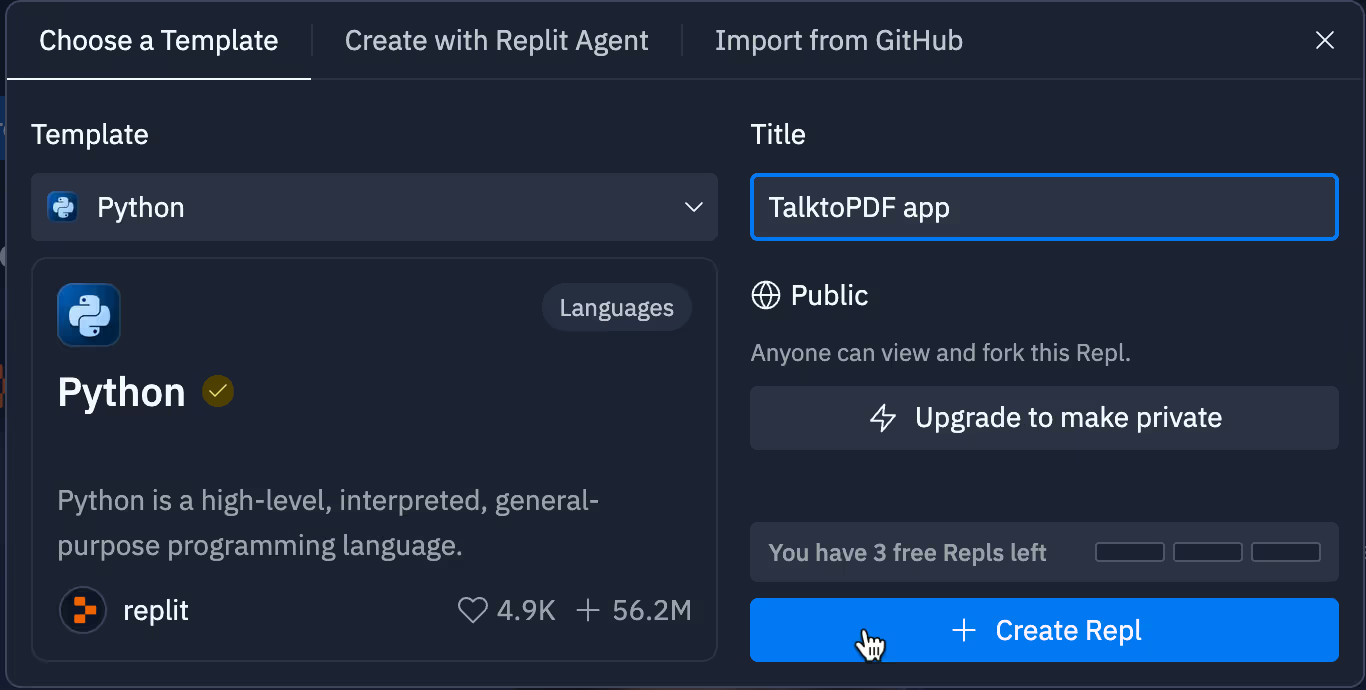
Since we were on the free account, we decided to keep it public until we got another tutorial with Replit to write!
A code editor window opens up. The center panel is ready for some code action.
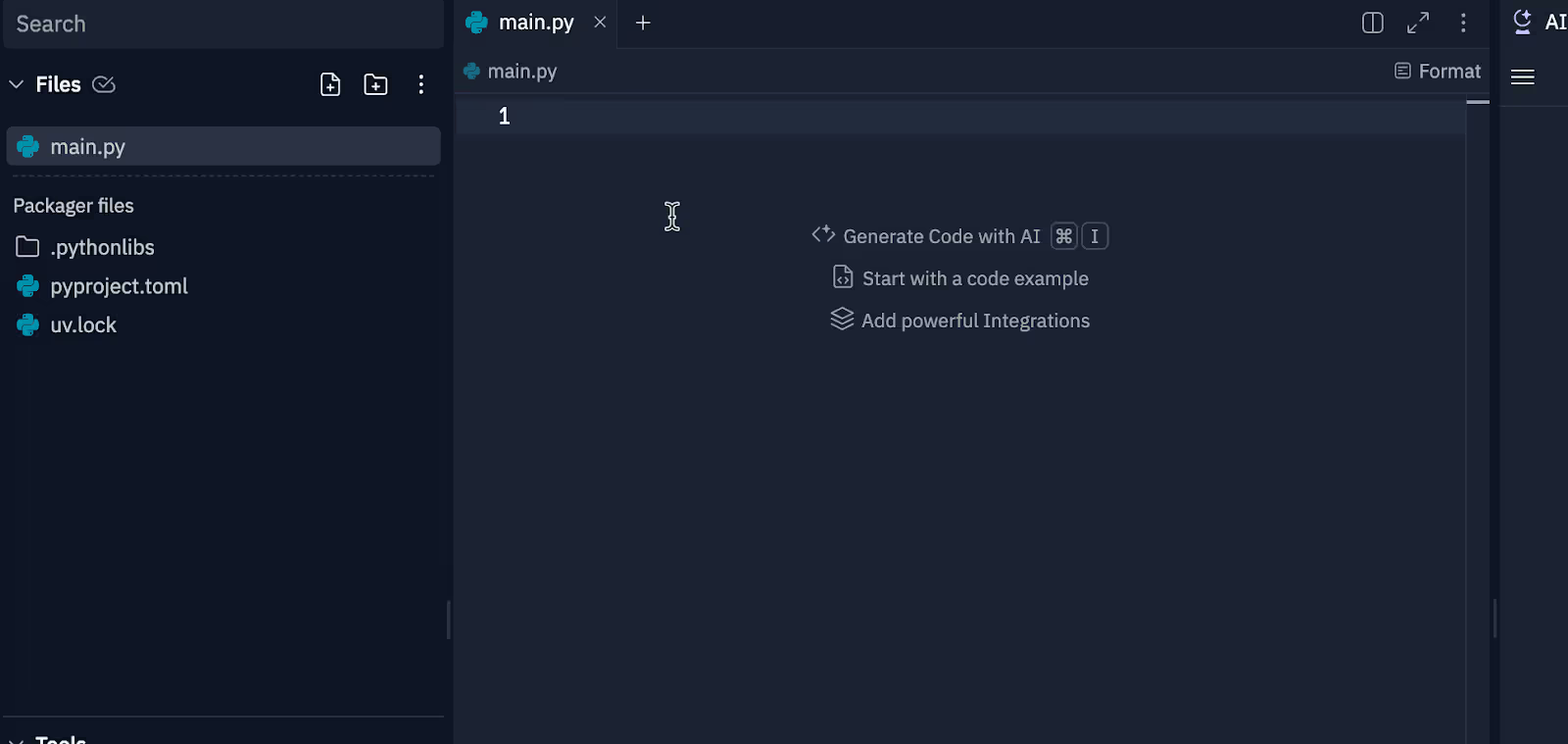
What you need to do is to install llamaIndex in Replit.
But before that, if you’re worried about code, a get-started guide on the LlamaInde website shows you how to build an app with 5 lines of code! We’ll be using it. Access the docs at https://docs.llamaindex.ai/en/stable/#getting-started.
However, let’s install LlamaIndex first. Then, switch the whole thing to ‘Shell’ by clicking the ‘+’ sign in the tab column and searching for ‘Shell.’

Type the following command and press Enter.
pip install llama-index
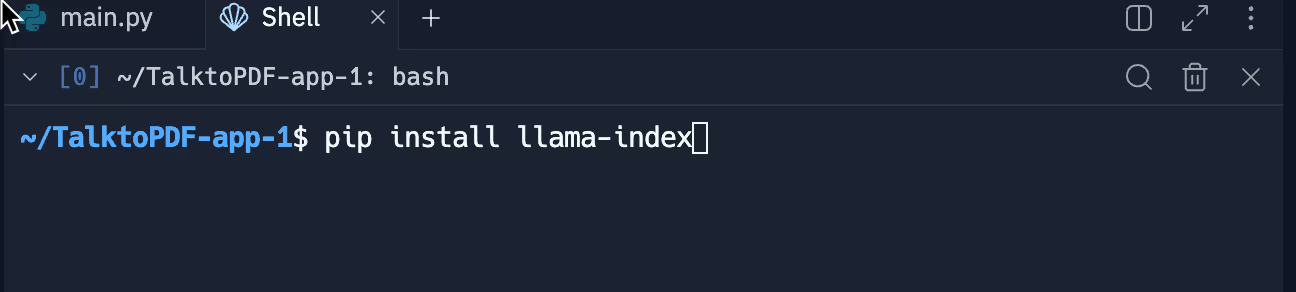
An unlimited amount of code will start appearing, looking something like a scene from the movie Matrix. This is Replit installing the LlamaIndex app into our little project.
Wait for 5-10 seconds to complete. It’ll look something like this when it’s done.

Did you open the LlamaIndex guide we asked you to open? Copy the following code:
from llama_index import VectorStoreIndex, SimpleDirectoryReader
documents = SimpleDirectoryReader("data").load_data()
index = VectorStoreIndex.from_documents(documents)
query_engine = index.as_query_engine()
response = query_engine.query("Some question about the data should go here")
print(response)
Paste it into the main.py tab in the Replit code window.
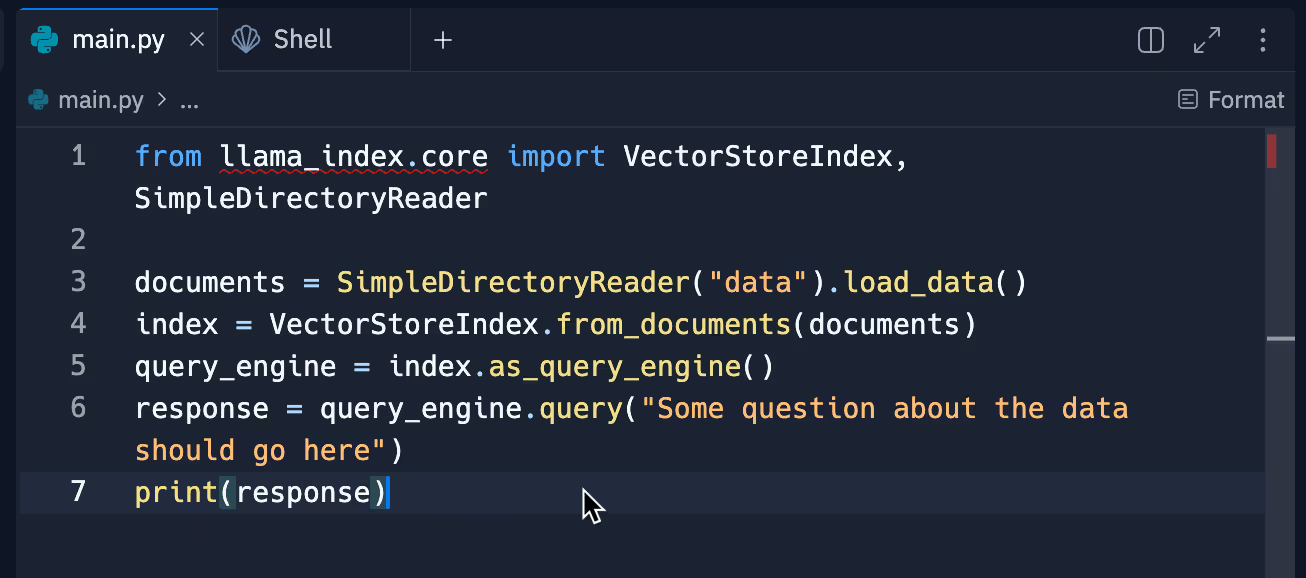
In the main tab section, click the ‘+’ sign. Find and click ‘Secrets.’

Go to platform.openapi.com and use your $10 credit to generate a secret API key for this little project of yours. Go to API keys in the left column and click the ‘Create’ a new secret key’ button to generate an API key.

Go back to the ‘Secrets’ tab you opened in Replit. Click ‘Add Secret’ and paste the key there. Name it OPENAI_API_KEY.
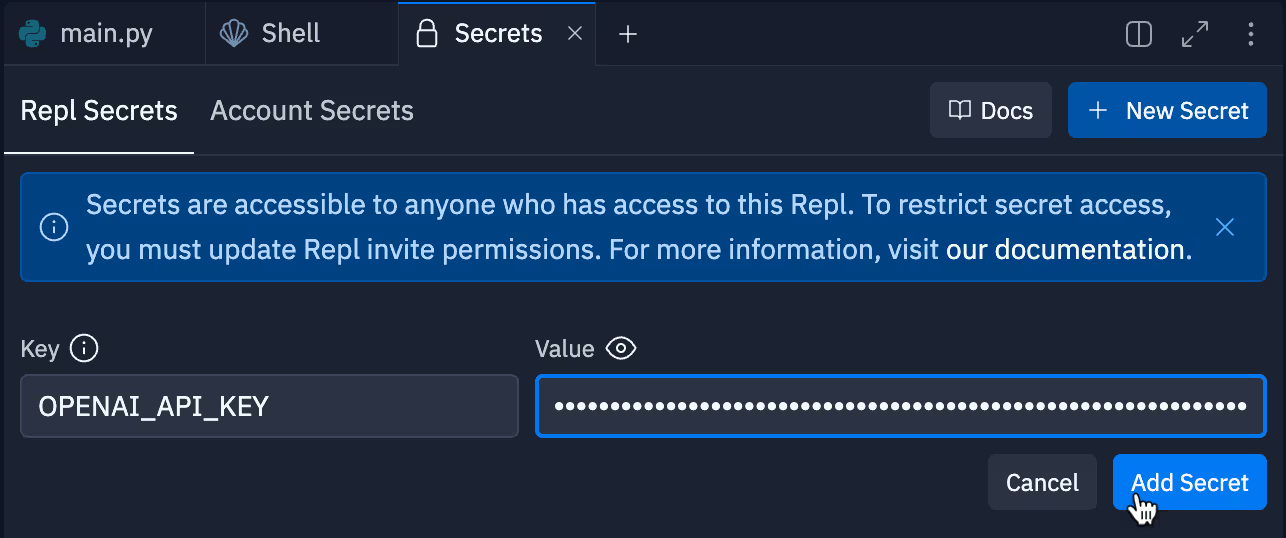
Go to the left column in Replit and create a new folder. Name it ‘Data.’
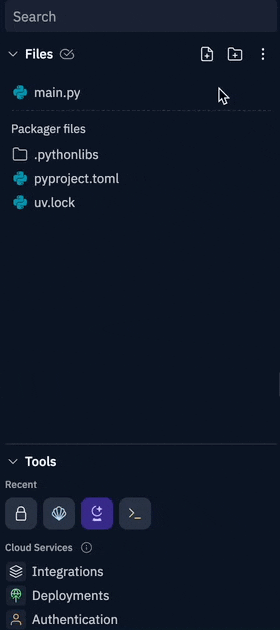
Since it is a talk-to-PDF app, we need a PDF to talk to! Upload a single or multiple PDF files in the folder you just created. You are not limited to PDF; you can upload .docx, .text, or any text-based document. If you are wondering how to upload a file since there is no upload button under the folder, you only need to drag and drop the file.
For our tutorial, I have downloaded a ‘Pool Care Guide’ PDF and uploaded it to the folder.
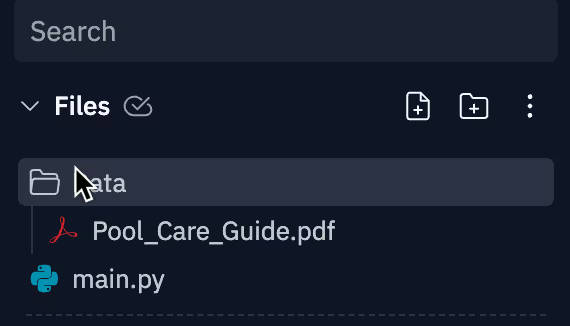
Click the main.py tab in the code console window. You will notice that the LlamaIndex code has been updated. You can see the code area where you can type some questions for the app to talk to the PDF.
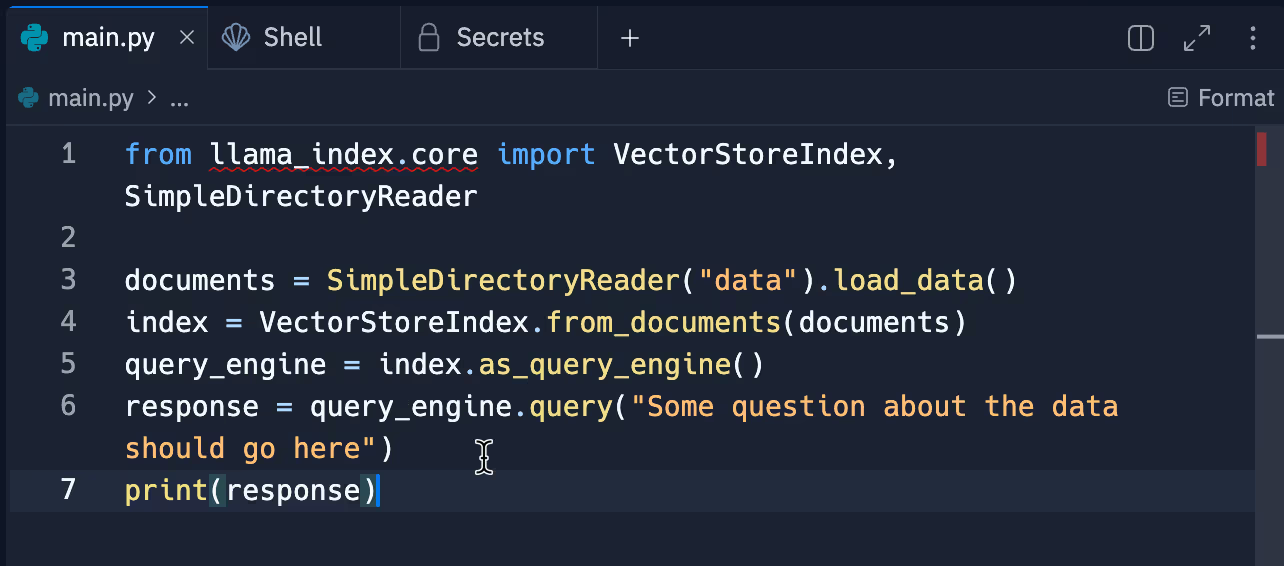
Type your question in the place where it says, “ Some questions about the data should go here.” We asked, ‘What is the best way to clean a pool filter?’

After typing the question, click the ’ Run’ button at the top of the console window. Then click the ‘Console’ tab in the right column window. The response will appear here.
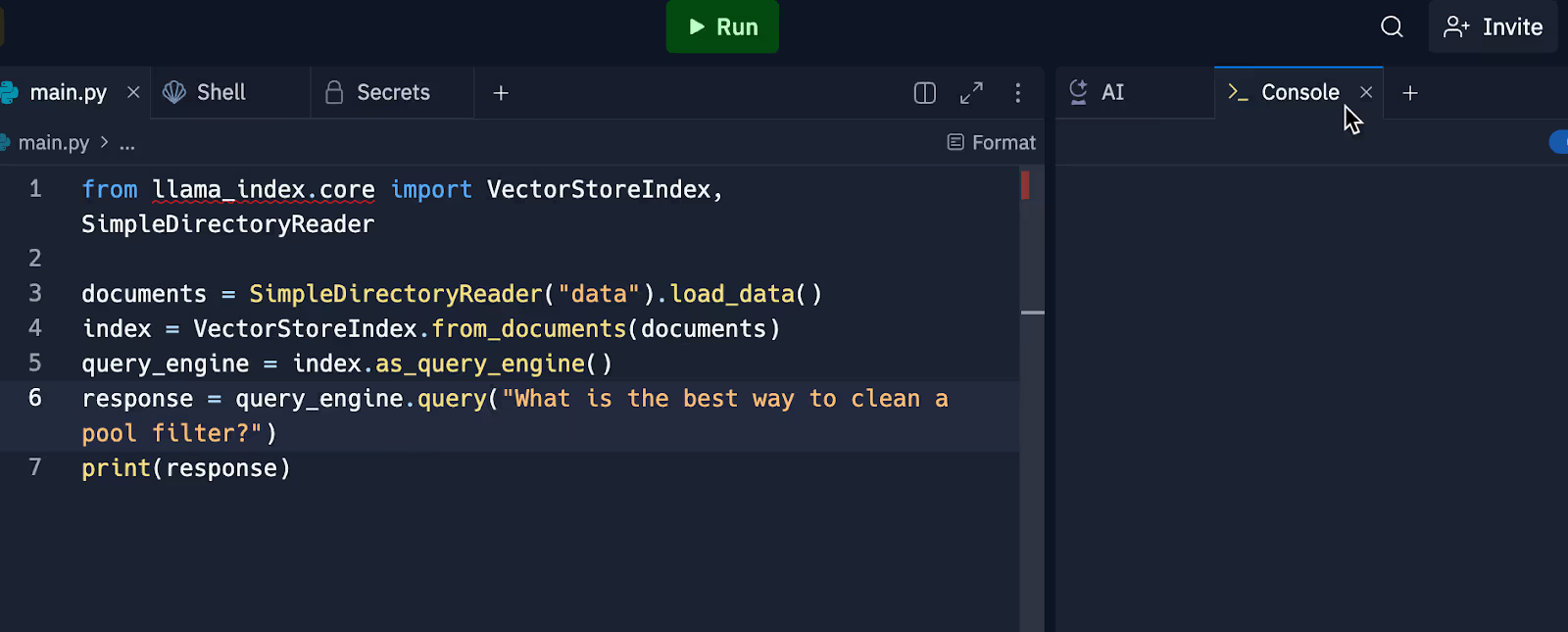
It will be blank for 5-10 seconds. In the background, LlamaIndex is parsing the document in the data folder. It is creating embeddings for them using the OpenAI API. The OpenAI is querying those embeddings in the data to answer our question.
In a few seconds, a response will appear. It will be a summarized answer.

That’s wonderful. It listed all the steps to clean a pool filter. We love it.
Step 2 - Use Replit's AI copilot
Going back to the code window and typing questions in between inverted commas is very inconvenient. Let’s ask Replit AI to generate a code that will allow us to input out questions in a good-looking query box every time we run the code.
Since we are not coders, we must rely on Replit Copilot AI. You can always go to your buddy ‘ChatGPT’ to generate a code. But I guess Replit AI might be more accurate.
Click the Replit AI tab in the right column window and use the following prompt:
Prompt:
Can you update the code so I can input the query every time it runs?
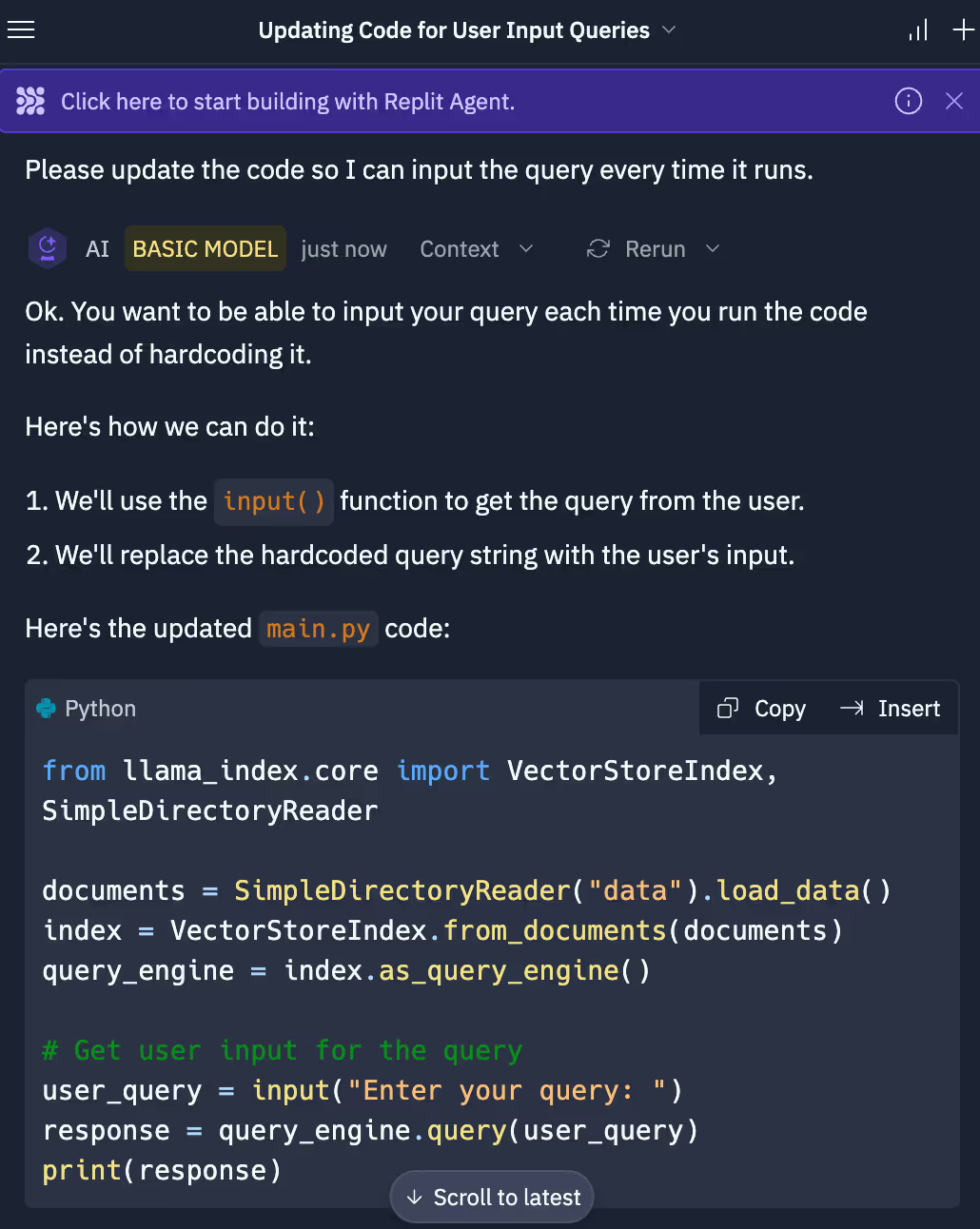
You can subscribe to Replit AI, and it will do all the steps mentioned in reply to your AI query. However, we are on a free model, so we have to do the steps ourselves.
Replace the code in the main.py with the code generated by AI.

It’s time to run the code to display the question input box.
Then, go ahead and run it. It will show a query box at the bottom of the console. You can write your question, and it will answer it.

There you go. Enter your query, and it will answer it. For example, we asked how to clean the Salt Chlorinator Cell. Press Enter.
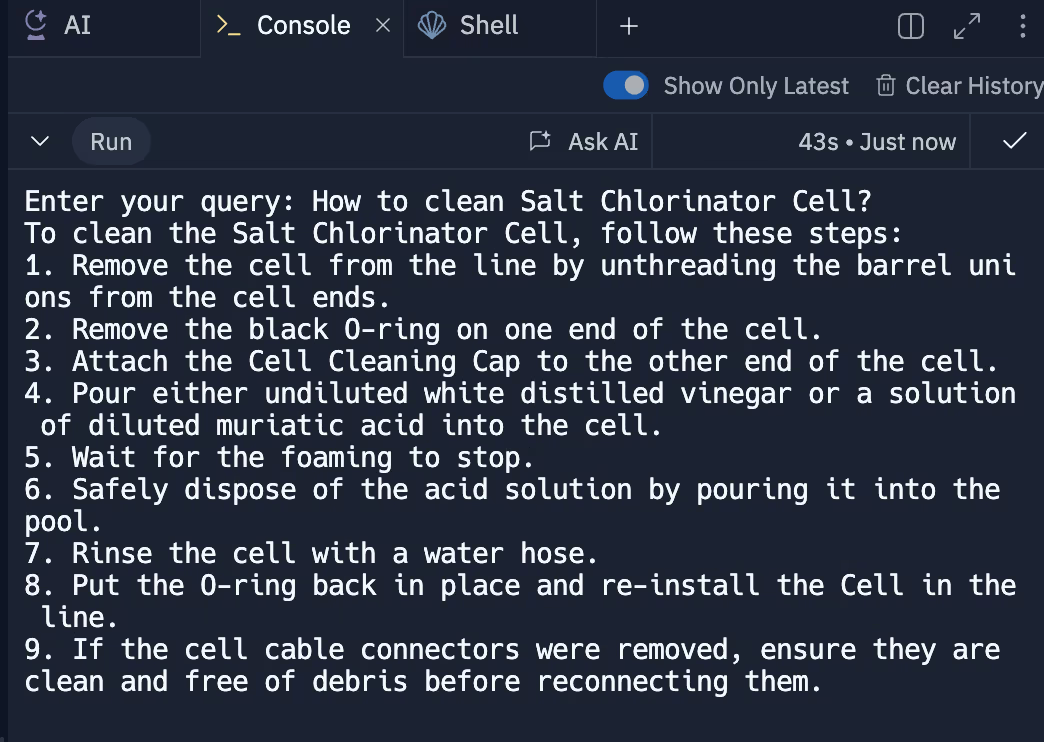
After a few seconds, it generated an accurate answer.
Step 3 - Design a web interface for the app
Running all the code and generating an answer is pretty hard. What if we could design a web interface for the app so it can answer questions promptly?
How about asking Replit AI to turn this into a small app? Let’s do it.
Go to the Replit AI tab and paste the following prompt:
Prompt
Can we create a simple web app that allows me to manage and view the files in the "data" folder, submit a query, view the response, and reset for a new query?
This is what it generated.
from flask import Flask, request, render_template_string, redirect, url_for
import os
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader
app = Flask(__name__)
UPLOAD_FOLDER = 'data'
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
user_query = request.form.get('query')
if user_query:
documents = SimpleDirectoryReader(UPLOAD_FOLDER).load_data()
index = VectorStoreIndex.from_documents(documents)
query_engine = index.as_query_engine()
response = query_engine.query(user_query)
return render_template_string(TEMPLATE, files=os.listdir(UPLOAD_FOLDER), response=response)
return render_template_string(TEMPLATE, files=os.listdir(UPLOAD_FOLDER))
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return redirect(url_for('index'))
file = request.files['file']
if file.filename == '':
return redirect(url_for('index'))
if file:
file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename))
return redirect(url_for('index'))
@app.route('/reset', methods=['POST'])
def reset():
response = ''
return render_template_string(TEMPLATE, files=os.listdir(UPLOAD_FOLDER), response=response)
TEMPLATE = """
<!doctype html>
<title>Query App</title>
<h1>Upload new File</h1>
<form method=post action="/upload" enctype=multipart/form-data>
<input type=file name=file>
<input type=submit value=Upload>
</form>
<h2>Available Files</h2>
<ul>
{% for file in files %}
<li>{{ file }}</li>
{% endfor %}
</ul>
<h2>Enter Query</h2>
<form method=post>
<input type=text name=query>
<input type=submit value="Submit Query">
</form>
{% if response %}
<h2>Response</h2>
<p>{{ response }}</p>
<form method=post action="/reset">
<input type=submit value="Reset">
</form>
{% endif %}
"""
if __name__ == '__main__':
os.makedirs(UPLOAD_FOLDER, exist_ok=True)
port = int(os.environ.get("PORT", 5000))
app.run(host='0.0.0.0', port=port, debug=True)
Replace the code in the main.py with this code. Run it to generate a web app that will allow us to ask questions related to the PDF we uploaded in the first step.
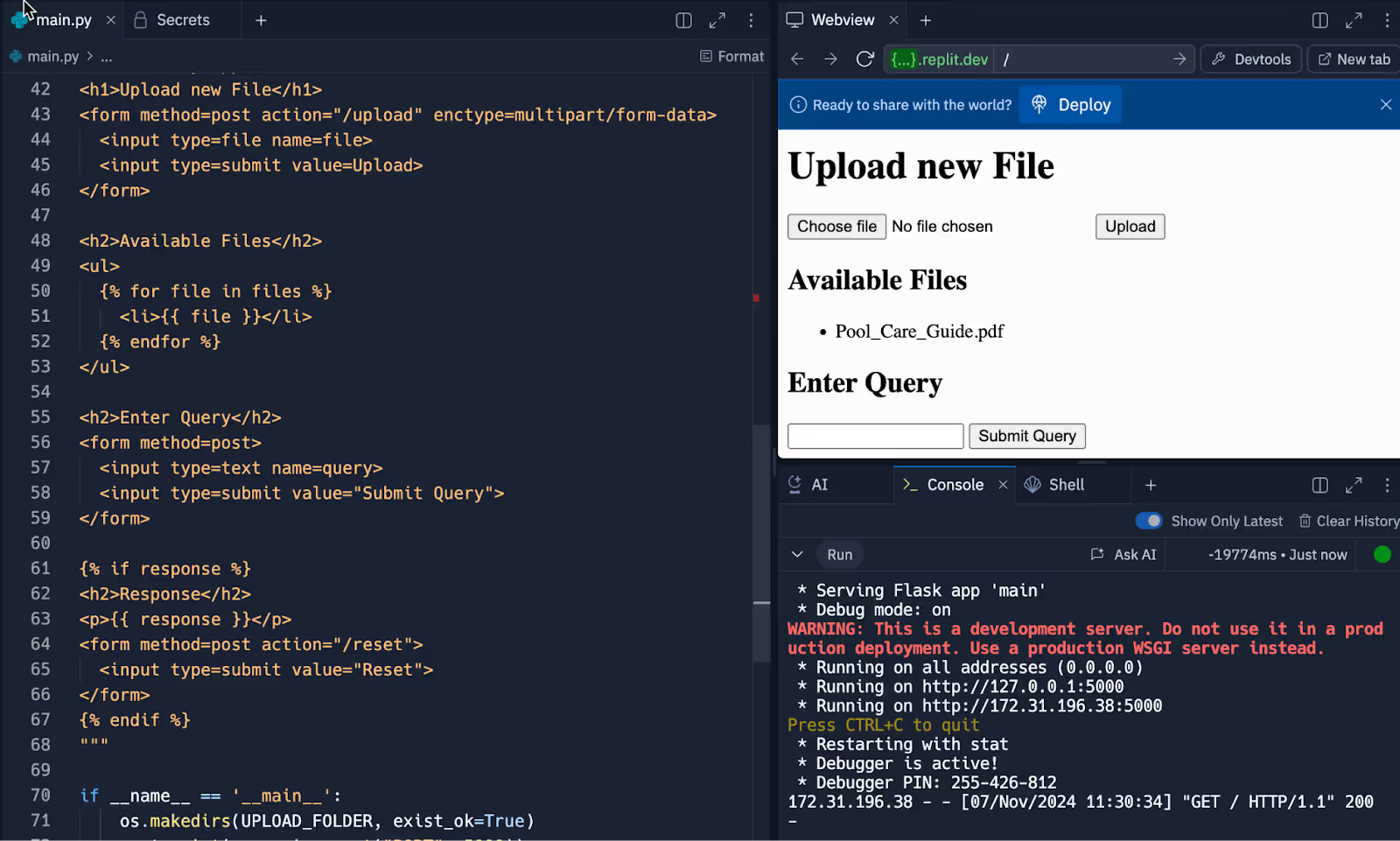
It’s basic, but it will do the job.
Let’s test it. Upload another file using the web app upload button. We chose a PDF that explains how to maintain a wooden floor. You can download it at: https://www.castlebri.com/wp-content/uploads/WoodFloorMaintenance.pdf

Choose a file and click Upload.

Yay! It’s uploaded. Let’s check our data folder to see if the file is there.

That’s looking great. Let’s ask a question using the web app we created.

Click ‘Submit Query.’
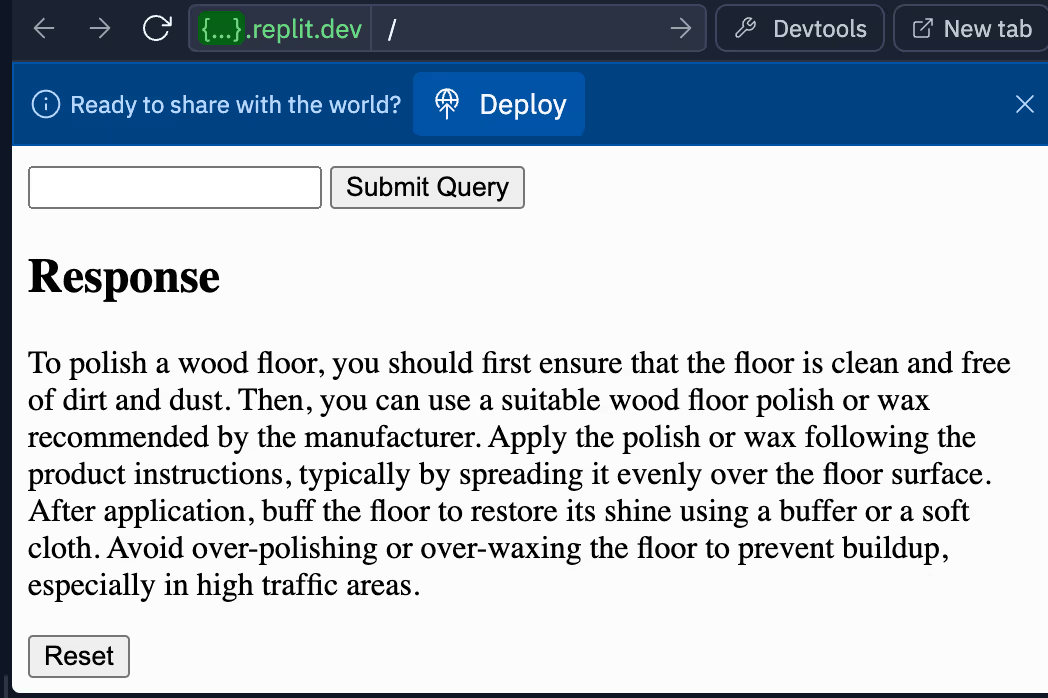
That turned out pretty good. You can upload multiple files and ask questions. It will read the PDFs and give you the answer.
If you've been following this guide, you might ask: can I just upload a PDF to a chatbot like ChatGPT and ask questions about it? Yes, that's possible, but creating your own Replit app comes with some significant benefits:
- You only pay for using the OpenAI API, which is very affordable and more budget-friendly than ChatGPT Plus or Claude Pro.
- You have complete control over the app and can adjust it to meet your needs.
- You can store and manage a collection of documents in the "data" folder.
- You can search through multiple documents at once without re-uploading them for each new conversation.
- You can design the interface precisely the way you want.
- You can add custom features that are tailored to your specific requirements.